Delta Lakes: Part 1 - When to Use Them, Benefits, and Challenges
Delta Lakes combine the scalability of data lakes with ACID transactions and schema enforcement, addressing issues like inconsistent data and complex updates. This post explores when to use Delta Lakes, their benefits, and the challenges you might face integrating them into your architecture.
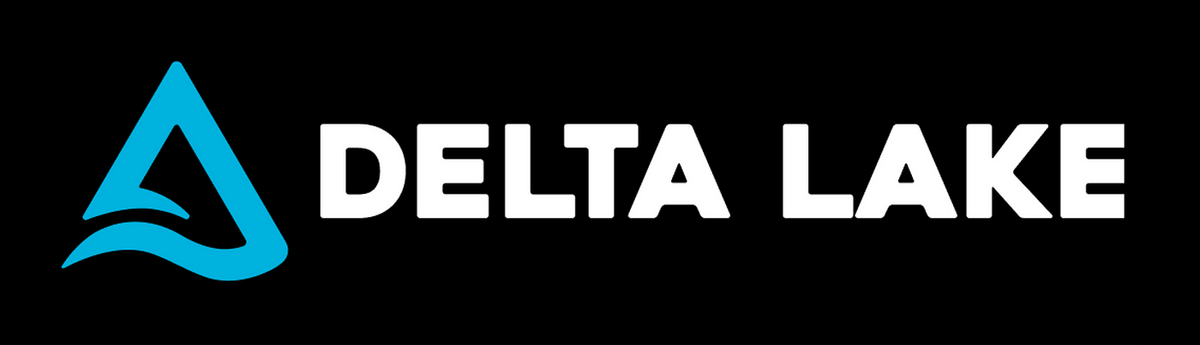
Introduction
In today’s data-driven world, businesses generate vast amounts of data from diverse sources, ranging from transactional systems and IoT devices to social media platforms. Storing and managing this data effectively is critical to unlocking its potential for analytics, reporting, and machine learning.
Enter the Data lake — a flexible and cost-efficient solution that allows organizations to store raw data in its native format. While data lakes provide scalability and flexibility, they also introduce challenges such as data reliability, query performance, and governance. Over time, these challenges can turn a data lake into a disorganized "data swamp," making it difficult to extract value and many more issues.
This is where Delta Lake comes in. But first things first.
What Is a Data Lake?
Before talking about Delta Lakes it is crucial to understand what is a Data Lake, because Delta Lakes exist on top of Data Lakes.
A data lake is a centralized repository that allows organizations to store vast amounts of structured, semi-structured, and unstructured data in its raw format. Unlike traditional databases or data warehouses, which store data in predefined schemas, a data lake adopts a schema-on-read approach, meaning data is only structured when it's accessed or processed.
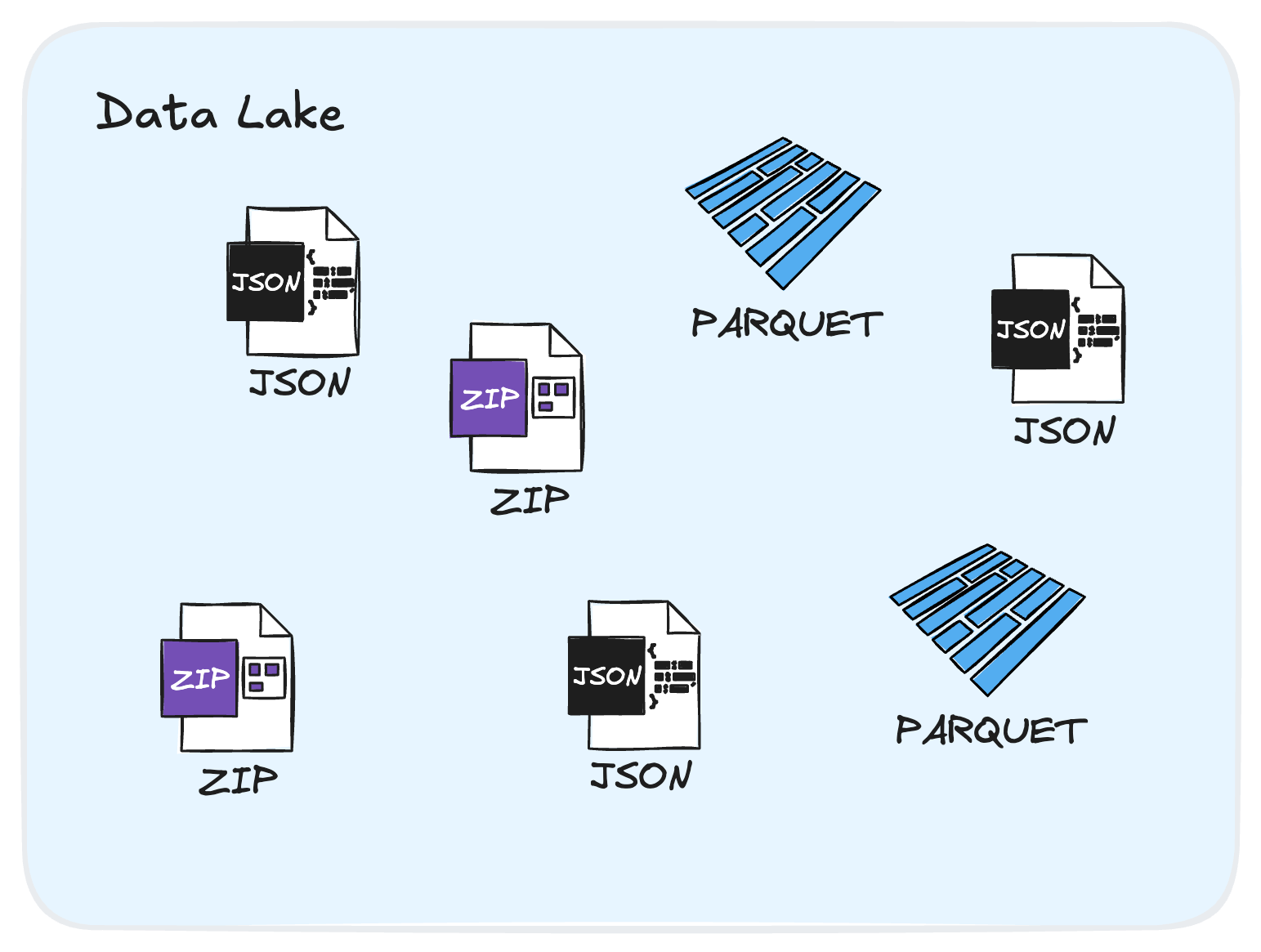
A Data Lake is a centralized repository that allows organizations to store vast amounts of structured, semi-structured, and unstructured data in its raw format.
Data Lakes are schema-on-read, meaning data is only structured when it's accessed or processed.
Key characteristics of a Data Lake:
- Raw Data Storage: Data is ingested in its native form without transformation, enabling flexibility for future use cases.
- Scalability: Designed to handle large volumes of data, often using distributed storage systems like Hadoop HDFS, Amazon S3, or Azure Blob Storage.
- Diverse Data Types: Supports structured (databases, spreadsheets), semi-structured (JSON, XML), and unstructured data (images, videos, logs).
- Accessibility: Enables a wide range of users (data scientists, analysts, engineers) to perform exploratory analytics using various tools.
- Cost-Efficiency: Often cheaper to store data in its raw form compared to processed formats used in traditional data warehouses.
Limitations of Traditional Data Lakes
While data lakes offer scalability and flexibility, they face several challenges that can hinder their usability and performance. Here are common issues encountered in traditional data lakes, along with illustrative code examples to demonstrate these pitfalls:
1. Data Consistency (Partial writes)
ISSUE: The ingestion pipeline may fail midway through execution, potentially corrupting the data lake with partially written data.
Example:
import pandas as pd
# Simulate writing data to a file in a data lake
try:
data = {"id": [1, 2, 3], "name": ["Alice", "Bob", "Charlie"]}
df = pd.DataFrame(data)
df.to_csv("data_lake/raw_data.csv", index=False)
# Simulate an error during the process
raise Exception("Pipeline failure")
except Exception as e:
print(f"Error: {e}")
# Data is partially written or corrupted your code here
Partially written data often requires manual cleanup, which can be cumbersome when dealing with large volumes of data streamed directly into a data lake. In such cases, you may not have enough time to respond quickly.
2. Lack of Schema Enforcement
ISSUE: Data lakes do not enforce schemas, leading to inconsistent or incorrect data formats.
Example:
import json
# Simulating appending inconsistent data
with open("data_lake/raw_data.json", "a") as f:
json.dump({"id": 1, "name": "Alice"}, f)
f.write("\n")
json.dump({"id": 2, "name": 42}, f) # Incorrect schema (name should be a string)
f.write("\n")
In this example, one dataset expects the name
field to be a string (e.g., "Alice"), while another incorrectly assumes it to be a number (e.g., 42). Such data corruption can disrupt downstream pipelines.
3. Concurrent Write Issues
ISSUE: Multiple processes writing to the same file simultaneously can cause conflicts or corrupt data.
Example:
import threading
def write_data(file_name, data):
with open(file_name, "a") as f:
f.write(data + "\n")
threads = [
threading.Thread(target=write_data, args=("data_lake/log.txt", "Write from Thread 1")),
threading.Thread(target=write_data, args=("data_lake/log.txt", "Write from Thread 2")),
]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
The resulting file may contain garbled or overlapping lines due to concurrent writes.
4. Slow Query Performance
ISSUE: Without indexing or optimization, querying large datasets in a data lake can be extremely slow.
Example:
import pandas as pd
# Simulating a large dataset
df = pd.DataFrame({"id": range(1, 1000001), "value": range(1000000, 0, -1)})
# Query for specific records
result = df[df["id"] == 999999]
print(result)
As the dataset grows, the time to scan the entire file increases linearly, making large-scale queries impractical.
5. Data Lineage and Versioning
ISSUE: Data lakes lack native support for tracking changes or versions, making it difficult to trace or reproduce historical states of data.
Example:
# Overwriting data without version tracking
with open("data_lake/sales_data.csv", "w") as f:
f.write("id,sales\n1,100\n")
# Update without preserving the original data
with open("data_lake/sales_data.csv", "w") as f:
f.write("id,sales\n1,150\n")
The original data is overwritten, and there’s no way to trace the changes or revert to the previous state.
6. Data Governance and Compliance
ISSUE: Enforcing compliance (e.g., GDPR's "right to be forgotten") in a data lake is manual and error-prone.
Example:
import pandas as pd
# Simulating manual deletion of a record
df = pd.read_csv("data_lake/users.csv")
df = df[df["user_id"] != 123] # Remove user 123 manually
df.to_csv("data_lake/users.csv", index=False)
Without ACID guarantees, concurrent operations might reintroduce deleted records or leave the system in an inconsistent state.
What is a Delta Lake
Delta Lake is an open-source storage layer that enhances traditional data lakes by making them more reliable, consistent, and performant. It ensures your data is trustworthy and easily accessible for analytics and machine learning. Think of it as a smarter data lake that combines the flexibility of a data lake with the reliability of a data warehouse.
Delta Lake is a storage layer that enhances traditional data lakes that combines the flexibility of a data lake with the reliability of a data warehouse
Delta Lake enhances traditional data lakes by making them more reliable, consistent, and performant.
Delta Lake was developed by Databricks, the creators of Apache Spark, to address the limitations of traditional data lakes. It’s an open-source project built on top of Apache Spark, making it seamlessly integrate with Spark-based workflows. Since its release, it has become a core part of the Lakehouse architecture, which combines the scalability of data lakes with the reliability of data warehouses.
Delta Lake uses a schema-on-write approach, meaning data must adhere to a predefined schema when it is written to a Delta Table. This ensures that the data stored in the table is consistent and queryable without the risk of schema mismatches.
Delta Lake uses a schema-on-write approach, meaning data must adhere to a predefined schema when it is written to a Delta Table
Key characteristics of a Delta Lake:
- ACID Transactions: Guarantees data consistency and reliability even during concurrent reads and writes or system failures.
- Schema Enforcement: Ensures all data adheres to a predefined structure to prevent corruption.
- Time Travel: Enables access to previous versions of your data for debugging, auditing, or reproducing results.
- Performance Optimization: Provides faster query performance with techniques like indexing, data skipping, and caching.
Delta Lakes to the rescue?
Let’s take a closer look at the challenges associated with traditional Data Lakes and explore how Delta Lake effectively addresses these issues.
1. Data Consistency (Partial Writes)
Challenge: In traditional data lakes, pipeline failures during writes can leave behind incomplete or corrupted data, requiring manual cleanup.
Delta Lake Solution: Delta Lake ensures ACID transactions, which guarantee atomicity. If a write operation fails, Delta Lake automatically rolls back the transaction, preventing partial data from being committed and maintaining a consistent state in the table.
Example:
from pyspark.sql import SparkSession
spark = SparkSession.builder \
.appName("DeltaLakeExample") \
.config("spark.sql.extensions", "io.delta.sql.DeltaSparkSessionExtension") \
.config("spark.sql.catalog.spark_catalog", "org.apache.spark.sql.delta.catalog.DeltaCatalog") \
.getOrCreate()
try:
# Write initial data
df1 = spark.createDataFrame([{"id": 1, "name": "Alice"}])
df1.write.format("delta").mode("overwrite").save("/delta-lake/example")
# Simulate a pipeline failure
df2 = spark.createDataFrame([{"id": 2, "name": "Bob"}])
df2.write.format("delta").mode("append").save("/delta-lake/example")
raise Exception("Simulated failure!")
except Exception as e:
print(f"Error: {e}")
# Read data to confirm consistency
df = spark.read.format("delta").load("/delta-lake/example")
df.show()
2. Lack of Schema Enforcement
Challenge: Traditional data lakes often lack schema enforcement, allowing inconsistent or invalid data to be written, which can cause downstream processing issues.
Delta Lake Solution: Delta Lake enforces schemas at the time of writing, ensuring all data adheres to a predefined structure. It also supports schema evolution, allowing you to adapt your table schema as new requirements arise, without compromising the existing data integrity.
Example:
df1 = spark.createDataFrame([{"id": 1, "name": "Alice"}])
df1.write.format("delta").mode("overwrite").save("/delta-lake/schema-example")
# Attempt to append invalid data
try:
df_invalid = spark.createDataFrame([{"id": 2, "name": 42}]) # Invalid data type for 'name'
df_invalid.write.format("delta").mode("append").save("/delta-lake/schema-example")
except Exception as e:
print(f"Schema enforcement failed: {e}")
3. Concurrent Write Issues
Challenge: Concurrent writes in a data lake can lead to race conditions, data corruption, or conflicting updates.
Delta Lake Solution: Delta Lake’s ACID transactions and locking mechanism ensure isolation for concurrent writes. Multiple write operations can occur simultaneously without compromising data integrity, as Delta Lake handles them sequentially under the hood.
Example:
from delta.tables import DeltaTable
from threading import Thread
def append_data(data):
df = spark.createDataFrame(data)
df.write.format("delta").mode("append").save("/delta-lake/concurrent-example")
# Simulate concurrent writes
threads = [
Thread(target=append_data, args=([{"id": 1, "name": "Alice"}],)),
Thread(target=append_data, args=([{"id": 2, "name": "Bob"}],))
]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
# Verify the data
df = spark.read.format("delta").load("/delta-lake/concurrent-example")
df.show()
4. Slow Query Performance
Challenge: Querying large datasets in a data lake can be inefficient due to a lack of indexing, resulting in high latency and resource consumption.
Delta Lake Solution: Delta Lake optimizes performance with features like data skipping, Z-order indexing, and caching. These optimizations reduce the amount of data scanned during queries, significantly improving query speed and efficiency, especially for large-scale analytics.
Examples:
from pyspark.sql import SparkSession
from delta.tables import DeltaTable
# Initialize Spark session
spark = SparkSession.builder \
.appName("DeltaLakePerformance") \
.config("spark.sql.extensions", "io.delta.sql.DeltaSparkSessionExtension") \
.config("spark.sql.catalog.spark_catalog", "org.apache.spark.sql.delta.catalog.DeltaCatalog") \
.getOrCreate()
# Create a large dataset
data = [{"key": i, "value": 1000000 - i} for i in range(1, 1000001)]
df = spark.createDataFrame(data)
# Write data to Delta table
df.write.format("delta").mode("overwrite").save("/delta-lake/performance-example")
# Optimize the table using Z-order indexing on the 'key' column
delta_table = DeltaTable.forPath(spark, "/delta-lake/performance-example")
delta_table.optimize().executeZOrderBy("key")
# Query with optimized performance
result = spark.read.format("delta").load("/delta-lake/performance-example").filter("key = 999999")
result.show()
5. Data Lineage and Versioning
Challenge: Traditional data lakes do not natively support tracking changes or maintaining versions of the data, making it difficult to debug issues or reproduce past results.
Delta Lake Solution: Delta Lake provides time travel by storing snapshots of the data at every change. This allows users to access historical versions of the data, making it easier to debug, audit, or reproduce machine learning experiments.
Example:
# Write initial data
df1 = spark.createDataFrame([{"id": 1, "name": "Alice"}])
df1.write.format("delta").mode("overwrite").save("/delta-lake/versioning-example")
# Append new data
df2 = spark.createDataFrame([{"id": 2, "name": "Bob"}])
df2.write.format("delta").mode("append").save("/delta-lake/versioning-example")
# Query historical versions
df_v0 = spark.read.format("delta").option("versionAsOf", 0).load("/delta-lake/versioning-example")
df_v0.show()
6. Data Governance and Compliance
Challenge: Ensuring compliance, such as the GDPR’s "right to be forgotten," is challenging in data lakes, as deletions require manual intervention and are error-prone.
Delta Lake Solution: Delta Lake simplifies compliance with delete operations that are transactionally guaranteed. It ensures that sensitive data is fully removed from the table while maintaining consistency, making it easier to comply with regulations and audits.
Example:
from delta.tables import DeltaTable
# Write initial data
df = spark.createDataFrame([{"id": 1, "name": "Alice"}, {"id": 2, "name": "Bob"}])
df.write.format("delta").mode("overwrite").save("/delta-lake/governance-example")
# Transactionally delete data
delta_table = DeltaTable.forPath(spark, "/delta-lake/governance-example")
delta_table.delete("id = 1")
# Verify deletion
df = spark.read.format("delta").load("/delta-lake/governance-example")
df.show()
Real-World Examples
Delta Lake continues to be a leading data management solution in 2025, with numerous organizations leveraging its capabilities to enhance data reliability, performance, and scalability. Here are some notable examples:
- How Scribd Uses Delta Lake to Enable the World's Largest Digital Library
- Massive Data Processing in Adobe Experience Platform Using Delta Lake
Conclusion
In summary, Delta Lake provides a robust solution to many of the challenges that data lakes face. With its ACID transactions, schema enforcement, time travel, and performance optimization, Delta Lake brings reliability and consistency to a flexible, scalable data storage solution.
Delta Lake is ideal when you need:
- Reliable Data Pipelines: Ensures data consistency with ACID transactions, even during concurrent writes or failures.
- Use Case: Real-time analytics pipelines.
- Data Quality: Enforces schemas to maintain clean, consistent datasets.
- Use Case: Preparing data for machine learning or reporting.
- Time Travel: Tracks historical data for debugging, auditing, or reproducibility.
- Use Case: Reproducing ML experiments or querying past states.
- Concurrent Access: Handles multiple readers and writers safely with transaction isolation.
- Use Case: Shared analytics platforms.
- Faster Queries: Improves performance with data skipping and Z-order indexing.
- Use Case: Low-latency dashboards or interactive analytics.
- Unified Workloads: Supports batch and streaming data in the same table.
- Use Case: Real-time and batch processing pipelines.
- Compliance and Governance: Simplifies compliance with transactional deletes and audit trails.
- Use Case: GDPR and regulatory requirements.
Comparing Delta Lake and Traditional Data Lakes
To sum up, the table below outlines a direct comparison of their core features.
Feature | Data Lake | Delta Lake |
---|---|---|
Data Consistency | No ACID transactions; prone to partial writes and corruption. | Supports ACID transactions, ensuring consistent data even during failures. |
Schema Enforcement | No enforcement; allows unstructured, inconsistent data. | Enforces schemas at write time, ensuring clean and consistent data. |
Schema Evolution | Limited or manual changes; may cause downstream issues. | Supports schema evolution, allowing seamless updates to table schemas. |
Query Performance | Slow; no indexing or optimization. | Optimized with data skipping, caching, and Z-order indexing for faster queries. |
Historical Data Access | Not supported; changes overwrite previous data. | Provides time travel, enabling access to previous data versions. |
Concurrent Writes | No isolation; race conditions may corrupt data. | Ensures isolation with ACID transactions, allowing safe concurrent writes. |
Batch & Streaming | Separate tools required; results in inconsistent data. | Unified support for batch and streaming workloads in a single table. |
Data Governance | Manual; challenging to delete or audit data. | Simplifies compliance with transactional deletes and audit logs. |
Debugging & Reproducibility | Difficult; no built-in version control. | Supports reproducibility with versioning and time travel. |
Cost Efficiency | Storage-focused but lacks reliability features. | Combines the scalability of data lakes with the reliability of data warehouses. |
In this post, we’ve explored the key features and benefits of Delta Lake and how it enhances traditional data lakes by introducing powerful capabilities. As data lakes continue to grow in popularity, understanding how Delta Lake addresses common challenges becomes crucial for building reliable, scalable data solutions.
In Part 2, we will dive deeper into the inner workings of Delta Lake. We’ll explore its architecture, how it achieves data consistency, and the mechanisms that make it such a powerful tool for managing large-scale data pipelines. Stay tuned as we uncover the technical details behind Delta Lake’s success and how you can leverage it in your own data workflows.